Web Development: JavaScript
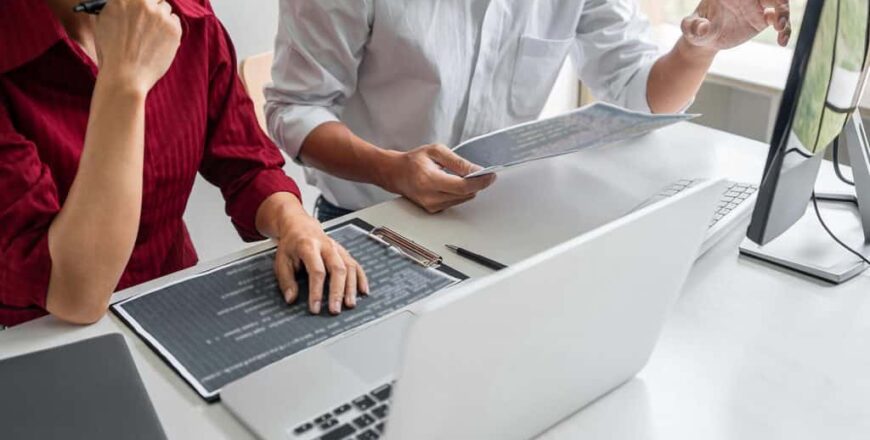
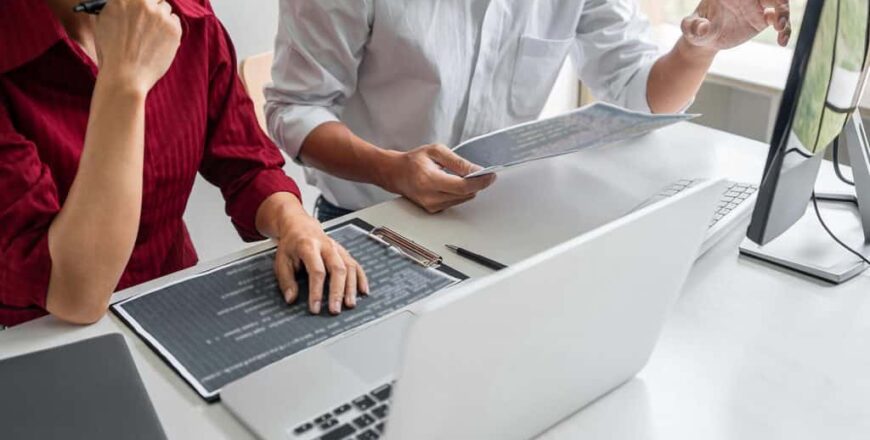
This JavaScript course is designed for individuals looking to become proficient in one of the most widely used programming languages for web development. Participants will embark on a comprehensive journey, starting from the basics of JavaScript and progressing to advanced concepts, frameworks, and best practices. The course will empower learners to build dynamic and interactive web applications.
Module 1. JavaScript: An Introduction
Module 2. The Basics
-
3Tools for JavaScript development(In progress)
Tools for JavaScript development(In progress)
-
4Introducing the browser console
Introducing the browser console
-
5Add inline JavaScript to a HTML document
Add inline JavaScript to a HTML document
-
6Add JavaScript in an external file
Add JavaScript in an external file
-
7How to write JavaScript: A crash course(In progress)
How to write JavaScript: A crash course(In progress)
Module 3. Working with data
-
8Variables: The catch-all containers of JavaScript
Variables: The catch-all containers of JavaScript
-
9Data types in JavaScript
Data types in JavaScript
-
10Arithmetic operators and math
Arithmetic operators and math
-
11Working with strings and numbers
Working with strings and numbers
-
12Conditional statements and logic
Conditional statements and logic
-
13Advanced conditions and logic
Advanced conditions and logic
-
14Arrays
Arrays
-
15Properties and methods in arrays
Properties and methods in arrays
Module 4. Functions and Objects
-
16Functions in JavaScript
Functions in JavaScript
-
17Build a basic function(In progress)
Build a basic function(In progress)
-
18Add arguments to the function
Add arguments to the function
-
19Return values from a function
Return values from a function
-
20Anonymous functions
Anonymous functions
-
21Immediately invoked functional expressions
Immediately invoked functional expressions
-
22Variable scope
Variable scope
-
23ES2015: let and const
ES2015: let and const
-
24Make sense of objects
Make sense of objects
-
25Object constructors
Object constructors
-
26Sidebar: Dot and bracket notation
Sidebar: Dot and bracket notation
-
27Closures
Closures
Module 5. JavaScript and the DOM, Part 1: Changing DOM Elements
-
28DOM: The document object model
DOM: The document object model
-
29Target elements in the DOM with querySelector methods
Target elements in the DOM with querySelector methods
-
30Access and change elements
Access and change elements
-
31Access and change classes
Access and change classes
-
32Add DOM elements
Add DOM elements
-
33Apply inline CSS to an element
Apply inline CSS to an element
Module 6. Project: Create an Analog Clock
-
34Create an analog clock: Project breakdown
Create an analog clock: Project breakdown
-
35Use CSS to move clock hands
Use CSS to move clock hands
-
36Use JavaScript to move clock hands
Use JavaScript to move clock hands
-
37Get the current hour, minute, and second with the Date() object
Get the current hour, minute, and second with the Date() object
-
38Show the current time using fancy math
Show the current time using fancy math
-
39Make the clock move forward second by second
Make the clock move forward second by second
-
40Solve the pesky "return to zero" problem
Solve the pesky "return to zero" problem
Module 7. JavaScript and the DOM, Part 2: Events
-
41What are DOM events?
What are DOM events?
-
42Some typical DOM events
Some typical DOM events
-
43Trigger functions with event handlers
Trigger functions with event handlers
-
44Add and use event listeners
Add and use event listeners
-
45Pass arguments via event listeners
Pass arguments via event listeners
Module 8. Project: Typing Speed Tester
-
46Rundown of HTML markup
Rundown of HTML markup
-
47Build a count-up timer
Build a count-up timer
-
48Detect spelling errors by matching strings
Detect spelling errors by matching strings
-
49Stop the timer when the test is done
Stop the timer when the test is done
-
50Add a reset button
Add a reset button
Module 9. Loops
Module 10. Project: Automated Responsive Images Markup
-
54Project breakdown
Project breakdown
-
55Rundown of project setup
Rundown of project setup
-
56Loop through all images in the document
Loop through all images in the document
-
57Create function to generate srcset value
Create function to generate srcset value
-
58Update img markup with srcset and sizes attributes
Update img markup with srcset and sizes attributes
Module 11. Troubleshooting, Validating, and Minifying JavaScript
-
59JavaScript validation and troubleshooting
JavaScript validation and troubleshooting
-
60Troubleshooting JavaScript
Troubleshooting JavaScript
-
61Send troubleshooting info to the console
Send troubleshooting info to the console
-
62Step through your JavaScript with browser tools
Step through your JavaScript with browser tools
-
63Online script linting
Online script linting
-
64Automate script linting
Automate script linting
-
65Automate script minification
Automate script minification
Module 12. Bonus Chapter: Ask the Instructor
-
66What are arrow functions?
What are arrow functions?
-
67What do I learn first? JavaScript? TypeScript?
What do I learn first? JavaScript? TypeScript?
-
68How do I write strings in ES2015?
How do I write strings in ES2015?
-
69What does the % symbol do?
What does the % symbol do?
-
70Why use the querySelector() and querySelectorAll() methods?
Why use the querySelector() and querySelectorAll() methods?